Software Design for "Intelligent Greenhouse System ", codes.
- carlche
- 2016年2月29日
- 讀畢需時 13 分鐘
In the following place I am going to post my codes for this software in the order of:
#0.AchartEngine plotting program for spectrum line
#1.login page/ landing interface
#2.greenhouse page/ greenhouse interface (for next step--node)
#3.node page/ node interface (for next step--real time)
#4.real time parameter/ ...interface
#5.searching page/searching page interface
#6.spectrum searching/ display/ intercace
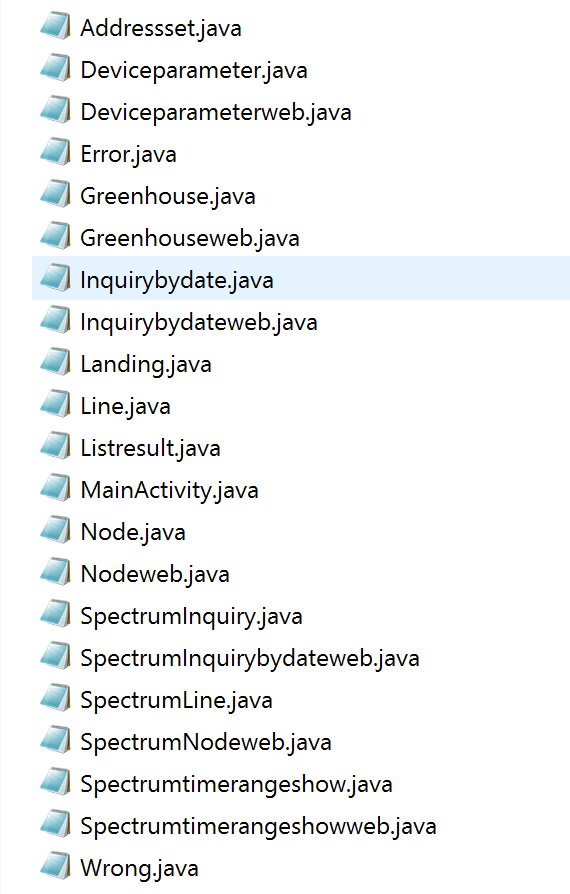
#0. AchartEngine plotting program for spectrum line
*************************************************************************
package com.example.iot;
import org.achartengine.ChartFactory; import org.achartengine.model.XYMultipleSeriesDataset; import org.achartengine.model.XYSeries; import org.achartengine.renderer.XYMultipleSeriesRenderer; import org.achartengine.renderer.XYSeriesRenderer;
import android.app.Activity; import android.content.Intent; import android.graphics.Color; import android.graphics.Paint.Align; import android.os.Bundle;
public class SpectrumLine extends Activity {
private String confirmedname; private String greenhouse; private String node; private String time;
private String[] getpara; private String[] wavelength; private Double[] wavelengthset; private String[] ref; private Double[] refset;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.spectrumline);
Intent intent = getIntent(); confirmedname = intent.getStringExtra("username"); greenhouse = intent.getStringExtra("greenhouse"); node = intent.getStringExtra("node"); time = intent.getStringExtra("time");
SpectrumInquirybydateweb siqb = new SpectrumInquirybydateweb( confirmedname, greenhouse, node, time); System.out.println(confirmedname+greenhouse+node+time); Thread t6 = new Thread(siqb); t6.start(); while (siqb.tag) {
} if(siqb.length==0) { Intent intenterror = new Intent(); intent.setClass(SpectrumLine.this, Error.class); SpectrumLine.this.startActivity(intent); } else { //杅擂煦賃 getpara=siqb.listcontent[0].split("\\s+"); for(int x=0;x<getpara.length-1;x++) { System.out.println(getpara[x]); } //杅擂煦郪 ref = new String[getpara.length/ 2]; wavelength = new String[getpara.length/ 2];
for (int x = 0; x <= (getpara.length / 2 - 1); x++) { wavelength[x] = getpara[2 * x]; ref[x] = getpara[2 * x + 1]; } wavelengthset = new Double[wavelength.length]; refset = new Double[ref.length];
for (int x = 0; x <= ref.length - 1; x++) { refset[x] = Double.parseDouble(ref[x]); if(refset[x]>2) refset[x]=2.0; else if (refset[x]<-1) refset[x]=-1.0; }
for (int x = 0; x <= wavelength.length - 1; x++) { wavelengthset[x] = Double.parseDouble(wavelength[x]); } System.out.println(wavelengthset.length); System.out.println(refset.length);
XYMultipleSeriesRenderer renderer = new XYMultipleSeriesRenderer(); XYMultipleSeriesDataset dataset = new XYMultipleSeriesDataset(); renderer.setZoomButtonsVisible(false); renderer.setYAxisMin(-30); renderer.setYAxisMax(30); renderer.setYAxisMin(-1); renderer.setYAxisMax(2); renderer.setApplyBackgroundColor(true); renderer.setBackgroundColor(Color.BLACK); renderer.setShowGrid(true); renderer.setYTitle("毀扞掀/%"); renderer.setXTitle("疏酗/nm"); renderer.setLabelsColor(Color.CYAN); renderer.setAxisTitleTextSize(20); renderer.setLabelsTextSize(25); renderer.setYLabels(20); renderer.setXLabels(40); renderer.setPanEnabled(true, false); renderer.setXLabelsAngle(300); renderer.setShowLegend(true);
XYSeries spseries = new XYSeries(" ");
renderer.setXLabelsAlign(Align.RIGHT); dataset.addSeries(spseries);
for(int i=0; i<ref.length-1;i++) { spseries.add(wavelengthset[i], refset[i]); } XYSeriesRenderer xyRenderer1 = new XYSeriesRenderer(); xyRenderer1.setColor(Color.CYAN); xyRenderer1.setDisplayChartValues(false); xyRenderer1.setLineWidth(2); renderer.addSeriesRenderer(xyRenderer1);
Intent spline = ChartFactory.getLineChartIntent(this, dataset, renderer); startActivity(spline);
} }
}
#1.login page/ landing interface
**********************************************************************************
package com.example.iot;
import android.app.Activity; import android.content.Context; import android.content.Intent; import android.content.SharedPreferences; import android.content.SharedPreferences.Editor; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.CheckBox; import android.widget.EditText; import android.widget.TextView;
public class MainActivity extends Activity { private Button button11 = null; private EditText edittext11 = null; private EditText edittext12 = null; private TextView show = null; private CheckBox passwordcheckbox=null; public SharedPreferences sp; private String name; private String password; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); show = (TextView) findViewById(R.id.show); passwordcheckbox=(CheckBox)findViewById(R.id.passwordcheckbox); sp = this.getSharedPreferences("userInfo", Context.MODE_WORLD_READABLE); edittext11 = (EditText) findViewById(R.id.edittext11); edittext12 = (EditText) findViewById(R.id.edittext12); button11 = (Button) findViewById(R.id.button11); button11.setOnClickListener(new Buttonlistener());
edittext11.setText(sp.getString("USER_NAME", "")); edittext12.setText(sp.getString("PASSWORD", "")); passwordcheckbox.setChecked(true); }
class Buttonlistener implements View.OnClickListener { @Override public void onClick(View v) { name = edittext11.getText().toString(); password = edittext12.getText().toString(); Landing ld = new Landing(name, password); Thread t1 = new Thread(ld); t1.start(); while (ld.tag) {
} //show.setText(ld.result); if (ld.result.equals("true")) { Intent intent = new Intent(); intent.putExtra("name", name); intent.setClass(MainActivity.this, Greenhouse.class); MainActivity.this.startActivity(intent);
} if (ld.result.equals("false")) {
edittext12.setError("渣昫腔蚚誧靡麼躇鎢"); edittext11.requestFocus(); edittext12.requestFocus();
} if (ld.result.equals("督昢籵陓祑都")) {
edittext12.setError("督昢籵陓祑都"); edittext11.requestFocus(); edittext12.requestFocus();
} //彆腎翹傖髡寀暮蛂梛瘍躇鎢 if(passwordcheckbox.isChecked()) { Editor editor = sp.edit(); editor.putString("USER_NAME", name); editor.putString("PASSWORD",password); editor.commit(); } if(!(passwordcheckbox.isChecked())) { Editor editor = sp.edit(); editor.putString("USER_NAME", ""); editor.putString("PASSWORD",""); editor.commit(); } }
}
} *******
Interface:
package com.example.iot;
import org.ksoap2.SoapEnvelope; import org.ksoap2.serialization.SoapObject; import org.ksoap2.serialization.SoapPrimitive; import org.ksoap2.serialization.SoapSerializationEnvelope; import org.ksoap2.transport.HttpTransportSE;
public class Landing implements Runnable { private static final String namespace = "http://landing"; private static final String methodname = "landing"; private static final String URL = "http://202.194.26.192:8080/landing/services/landing"; public String result =null; public boolean tag = true; private String username = null; private String userpassword = null;
Landing(String username, String userpassword) { this.username = username; this.userpassword = userpassword; }
public void run() { SoapObject rpc = new SoapObject(namespace, methodname); rpc.addProperty("userName", username); rpc.addProperty("userID", userpassword);
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope( SoapEnvelope.VER10); envelope.bodyOut = rpc; envelope.dotNet = true; envelope.setOutputSoapObject(rpc);
HttpTransportSE transport = new HttpTransportSE(URL);
try { // 覃蚚WebService transport.call(null, envelope); if (envelope.getResponse() != null) { org.ksoap2.serialization.SoapPrimitive soapPrimitive = (SoapPrimitive) envelope .getResponse(); result = soapPrimitive.toString();
} } catch (Exception e) { e.printStackTrace(); result="督昢籵陓祑都"; }
tag = false; }
}
#2.greenhouse page/ greenhouse interface (for next step--node)
page:
package com.example.iot;
import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.LinearLayout; import android.widget.TextView;
public class Greenhouse extends Activity {
private String confirmedname;
private TextView gt;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.greenhouse); gt = (TextView) findViewById(R.id.gt); Intent intent = getIntent(); confirmedname = intent.getStringExtra("name"); Greenhouseweb ghb = new Greenhouseweb(confirmedname); gt.setText("湮麟陓洘");
Thread t2 = new Thread(ghb); t2.start(); while (ghb.tag) {
} for (int j = 0; j <= ghb.length - 1; j++) {// 蔚鳳腔蜆蚚誧腔湮麟眕偌聽腔倛宒煦蹈狟懂ㄛ甜跤藩跺偌聽扢离偌瑩岈璃 LinearLayout greenlayout = (LinearLayout) findViewById(R.id.greenlayout); final Button t = new Button(this); t.setText(ghb.listcontent[j]); greenlayout.addView(t);
class Buttonlistener implements View.OnClickListener { @Override public void onClick(View v) { Intent intent = new Intent(); intent.putExtra("username", confirmedname); intent.putExtra("greenhouse", t.getText().toString()); intent.setClass(Greenhouse.this, Node.class); Greenhouse.this.startActivity(intent); } }
t.setOnClickListener(new Buttonlistener()); } } }
****
interface:
package com.example.iot;
import org.ksoap2.SoapEnvelope; import org.ksoap2.serialization.SoapObject; import org.ksoap2.serialization.SoapSerializationEnvelope; import org.ksoap2.transport.HttpTransportSE;
import android.widget.ListView;
public class Greenhouseweb implements Runnable { public ListView list; public int length; private SoapObject result; public String[] listcontent = new String[20]; private static final String namespace = "http://greenhouse"; private static final String methodname = "greenhouse"; private static final String URL = "http://202.194.26.192:8080/greenhouse/services/greenhouse"; public boolean tag = true; private String username = null;
Greenhouseweb(String username1) { this.username = username1; }
public void run() { SoapObject rpc = new SoapObject(namespace, methodname); rpc.addProperty("userName", username);
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope( SoapEnvelope.VER10); envelope.bodyOut = rpc; envelope.dotNet = true; envelope.setOutputSoapObject(rpc);
HttpTransportSE transport = new HttpTransportSE(URL);
try { // 覃蚚WebService transport.call(null, envelope); if (envelope.getResponse() != null) { result = (SoapObject) envelope.getResponse();
} } catch (Exception e) { e.printStackTrace(); }
length = result.getPropertyCount(); if (length > 0) { for (int i = 0; i <= length - 1; i++) { listcontent[i] = result.getProperty(i).toString(); }
} tag = false; }
}
#3.node page/ node interface (for next step--real time)
page:
package com.example.iot;
import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.LinearLayout; import android.widget.TextView;
public class Node extends Activity { private String confirmedname; private String greenhouse; private TextView nt;
private String[] spnodenum;
//蚚衾換菰統杅腔 @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.node); nt = (TextView) findViewById(R.id.nt);
Intent intent = getIntent(); this.confirmedname = intent.getStringExtra("username"); this.greenhouse = intent.getStringExtra("greenhouse"); nt.setText(greenhouse + "腔誹萸陓洘"); // 籵誹萸腔盄最 Nodeweb nb = new Nodeweb(confirmedname, greenhouse); Thread t2 = new Thread(nb); t2.start(); // 嫖誹萸腔盄最 SpectrumNodeweb snb = new SpectrumNodeweb(confirmedname, greenhouse); Thread t3 = new Thread(snb); t3.start(); while (nb.tag) { } while (snb.tag) { } // 扢离嫖誹萸腔偌聽眕摯綴哿魂雄 spnodenum=new String[snb.length]; for (int j = 0; j <= snb.length - 1; j++) { LinearLayout nodelayout = (LinearLayout) findViewById(R.id.nodelayout); final Button y = new Button(this); y.setText("覜眭誹萸"+snb.listcontent[j]+"瘍"); nodelayout.addView(y); spnodenum[j]=snb.listcontent[j]; final String df=spnodenum[j]; class Buttonlistener implements View.OnClickListener { @Override public void onClick(View v) { Intent intent = new Intent(); intent.putExtra("username", confirmedname); intent.putExtra("greenhouse", greenhouse); intent.putExtra("node",df); intent.setClass(Node.this, SpectrumInquiry.class); Node.this.startActivity(intent); } } y.setOnClickListener(new Buttonlistener());
}
// 扢离籵誹萸腔偌聽眕摯綴哿魂雄 for (int j = 0; j <= nb.length - 1; j++) { LinearLayout nodelayout = (LinearLayout) findViewById(R.id.nodelayout); final Button y = new Button(this); y.setText("誹萸"+nb.listcontent[j]+"瘍"); nodelayout.addView(y); final String nodenum=nb.listcontent[j];
class Buttonlistener implements View.OnClickListener { @Override public void onClick(View v) { Intent intent = new Intent(); intent.putExtra("username", confirmedname); intent.putExtra("greenhouse", greenhouse); intent.putExtra("node", nodenum); System.out.println(nodenum); intent.setClass(Node.this, Deviceparameter.class); Node.this.startActivity(intent); } } y.setOnClickListener(new Buttonlistener());
}
}
}
*****
interface:
package com.example.iot;
import org.ksoap2.SoapEnvelope; import org.ksoap2.serialization.SoapObject; import org.ksoap2.serialization.SoapSerializationEnvelope; import org.ksoap2.transport.HttpTransportSE;
import android.widget.ListView;
public class Nodeweb implements Runnable { public ListView list; public int length; public SoapObject result; public String[] listcontent = new String[20]; private static final String namespace = "http://node"; private static final String methodname = "node"; private static final String URL = "http://202.194.26.192:8080/node/services/node"; public boolean tag = true; private String username = null; private String greenhouse = null;
Nodeweb(String username1, String greenhouse1) { this.username = username1; this.greenhouse = greenhouse1; }
public void run() { SoapObject rpc = new SoapObject(namespace, methodname); rpc.addProperty("userName", username); rpc.addProperty("greenHouse", greenhouse);
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope( SoapEnvelope.VER10); envelope.bodyOut = rpc; envelope.dotNet = true; envelope.setOutputSoapObject(rpc);
HttpTransportSE transport = new HttpTransportSE(URL);
try {
transport.call(null, envelope); if (envelope.getResponse() != null) { result = (SoapObject) envelope.getResponse();
} } catch (Exception e) { e.printStackTrace(); }
length = result.getPropertyCount(); if (length > 0) { for (int i = 0; i <= length - 1; i++) { listcontent[i] = result.getProperty(i).toString();
}
} tag = false; }
}
#4.real time parameter/ ...interface
page:
package com.example.iot;
import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView;
public class Deviceparameter extends Activity { private String confirmedname; private String greenhouse; private String node; private TextView[] textset = new TextView[4]; private TextView[] timeset=new TextView[4]; private TextView[] device = new TextView[4]; private Button[] buttonset = new Button[4]; private String[] unit = { "⊥", "%RH", "Ppm", "Lux" }; private int j; private double tran1; private double tran2; private int tran3;
private TextView pt;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.deviceparameter); pt = (TextView) findViewById(R.id.pt); textset[0] = (TextView) findViewById(R.id.textset0); textset[1] = (TextView) findViewById(R.id.textset1); textset[2] = (TextView) findViewById(R.id.textset2); textset[3] = (TextView) findViewById(R.id.textset3); timeset[0]=(TextView) findViewById(R.id.timeset0); timeset[1]=(TextView) findViewById(R.id.timeset1); timeset[2]=(TextView) findViewById(R.id.timeset2); timeset[3]=(TextView) findViewById(R.id.timeset3);
device[0] = (TextView) findViewById(R.id.device0); device[1] = (TextView) findViewById(R.id.device1); device[2] = (TextView) findViewById(R.id.device2); device[3] = (TextView) findViewById(R.id.device3);
buttonset[0] = (Button) findViewById(R.id.buttonset0); buttonset[1] = (Button) findViewById(R.id.buttonset1); buttonset[2] = (Button) findViewById(R.id.buttonset2); buttonset[3] = (Button) findViewById(R.id.buttonset3);
Intent intent = getIntent(); this.confirmedname = intent.getStringExtra("username"); this.greenhouse = intent.getStringExtra("greenhouse"); this.node = intent.getStringExtra("node"); pt.setText("誹萸"+node+"瘍腔" + "腔遠噫陓洘");
Deviceparameterweb db = new Deviceparameterweb(confirmedname, greenhouse, node); Thread t2 = new Thread(db); t2.start();
while (db.tag) {
} //勤殿隙腔杅擂輛俴潼聆彆岆0麼氪10000ㄗ媼欬趙抯ㄘ寀珆尨峈※---§ 峈淏都杅擂寀珆尨 for (j = 0; j < textset.length; j++) { String mo = db.listcontent[j*2] + unit[j]; if((db.listcontent[j*2].equals("0"))||(db.listcontent[j*2].equals("10000.0"))) textset[j].setText("---"); else textset[j].setText(mo); } for (j = 0; j < timeset.length; j++) { if(db.listcontent[j*2+1].equals("0")) { String jo =db.listcontent[j*2+1]; timeset[j].setText("---"); } else { String[] ss1=db.listcontent[j*2+1].split("\\s+"); timeset[j].setText(ss1[1]); } }
buttonset[0].setOnClickListener(new Buttonlistener0()); buttonset[1].setOnClickListener(new Buttonlistener1()); buttonset[2].setOnClickListener(new Buttonlistener2()); buttonset[3].setOnClickListener(new Buttonlistener3()); }
class Buttonlistener0 implements View.OnClickListener {
public void onClick(View v) { Intent intent = new Intent();
intent.putExtra("username", confirmedname); intent.putExtra("greenhouse", greenhouse); intent.putExtra("node", node); intent.putExtra("device", device[0].getText()); intent.setClass(Deviceparameter.this, Inquirybydate.class); Deviceparameter.this.startActivity(intent); }
}
class Buttonlistener1 implements View.OnClickListener {
public void onClick(View v) { Intent intent = new Intent();
intent.putExtra("username", confirmedname); intent.putExtra("greenhouse", greenhouse); intent.putExtra("node", node); intent.putExtra("device", device[1].getText()); intent.setClass(Deviceparameter.this, Inquirybydate.class); Deviceparameter.this.startActivity(intent); }
}
class Buttonlistener2 implements View.OnClickListener {
public void onClick(View v) { Intent intent = new Intent();
intent.putExtra("username", confirmedname); intent.putExtra("greenhouse", greenhouse); intent.putExtra("node", node); intent.putExtra("device", device[2].getText()); intent.setClass(Deviceparameter.this, Inquirybydate.class); Deviceparameter.this.startActivity(intent); }
}
class Buttonlistener3 implements View.OnClickListener {
public void onClick(View v) { Intent intent = new Intent();
intent.putExtra("username", confirmedname); intent.putExtra("greenhouse", greenhouse); intent.putExtra("node", node); intent.putExtra("device", device[3].getText()); intent.setClass(Deviceparameter.this, Inquirybydate.class); Deviceparameter.this.startActivity(intent); }
}
}
********
interface:
package com.example.iot;
import java.math.BigDecimal;
import org.ksoap2.SoapEnvelope; import org.ksoap2.serialization.SoapObject; import org.ksoap2.serialization.SoapSerializationEnvelope; import org.ksoap2.transport.HttpTransportSE;
import android.widget.ListView;
public class Deviceparameterweb implements Runnable { public ListView list; public int length; public SoapObject result; public String[] listcontent = new String[20]; public BigDecimal[] listcontent2 = new BigDecimal[20]; private static final String namespace = "http://deviceparameter"; private static final String methodname = "deviceparameter"; private static final String URL = "http://202.194.26.192:8080/deviceparameter/services/deviceparameter"; public boolean tag = true; private String username = null; private String greenhouse = null; private String node = null;
Deviceparameterweb(String username1, String greenhouse1, String node1) { this.username = username1; this.greenhouse = greenhouse1; this.node = node1; }
public void run() { SoapObject rpc = new SoapObject(namespace, methodname); rpc.addProperty("userName", username); rpc.addProperty("greenHouse", greenhouse); rpc.addProperty("node", node);
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope( SoapEnvelope.VER10); envelope.bodyOut = rpc; envelope.dotNet = true; envelope.setOutputSoapObject(rpc);
HttpTransportSE transport = new HttpTransportSE(URL);
try {
transport.call(null, envelope); if (envelope.getResponse() != null) { result = (SoapObject) envelope.getResponse();
} } catch (Exception e) { e.printStackTrace(); }
length = result.getPropertyCount(); if (length > 0) { for (int i = 0; i <= length - 1; i++) { listcontent[i] = result.getProperty(i).toString(); }
} tag = false; }
}
#5.searching page/searching page interface
page:
package com.example.iot;
import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.AdapterView.OnItemSelectedListener; import android.widget.ArrayAdapter; import android.widget.Button; import android.widget.Spinner; import android.widget.TextView; import android.widget.TimePicker;
public class Inquirybydate extends Activity {
private String confirmedname; private String greenhouse; private String node; private String device;
private String sy; private String sm; private String sd; private String sh; private String smm; private String ey; private String em; private String ed; private String eh; private String emm; private String start; private String end; private String interval;
private String[] yearoption = { "2015", "2016", "2017" }; private String[] monthoption = { "01", "02", "03", "04", "05", "06", "07", "08", "09", "10", "11", "12" }; private String[] dayoption31 = { "01", "02", "03", "04", "05", "06", "07", "08", "09", "10", "11", "12", "13", "14", "15", "16", "17", "18", "19", "20", "21", "22", "23", "24", "25", "26", "27", "28", "29", "30", "31" }; public static ArrayAdapter<String> adapter1; public static ArrayAdapter<String> adapter2; public static ArrayAdapter<String> adapter3; public static ArrayAdapter<String> adapter4; public static ArrayAdapter<String> adapter5; public static ArrayAdapter<String> adapter6;
public int monthmark = 28; private Spinner startyear; private Spinner startmonth; private Spinner startday; private Spinner endyear; private Spinner endmonth; private Spinner endday; private TimePicker starttp; private TimePicker endtp;
private Button inquirydata; private Button inquirypicture; private TextView inquirytitle;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.inquirybydate); inquirytitle = (TextView) findViewById(R.id.inquirytitle); starttp = (TimePicker) findViewById(R.id.starttp); endtp = (TimePicker) findViewById(R.id.endtp); starttp.setIs24HourView(true); endtp.setIs24HourView(true);
Intent intent = getIntent(); this.confirmedname = intent.getStringExtra("username"); this.greenhouse = intent.getStringExtra("greenhouse"); this.node = intent.getStringExtra("node"); this.device = intent.getStringExtra("device"); inquirytitle.setText("偌桽脤戙" + device + "腔砆牉陓洘");
// 羲宎 爛爺狟嶺蹈桶 startyear = (Spinner) findViewById(R.id.startyear); adapter1 = new ArrayAdapter<String>(this, android.R.layout.simple_spinner_item, yearoption); adapter1.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item); startyear.setAdapter(adapter1); startyear.setVisibility(View.VISIBLE); startyear.setOnItemSelectedListener(new SpinnerSelectedListener1()); // 羲宎堎爺狟懂蹈桶 startmonth = (Spinner) findViewById(R.id.startmonth); adapter2 = new ArrayAdapter<String>(this, android.R.layout.simple_spinner_item, monthoption); adapter2.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item); startmonth.setAdapter(adapter2); startmonth.setVisibility(View.VISIBLE); startmonth.setOnItemSelectedListener(new SpinnerSelectedListener2()); // 羲宎狟嶺蹈桶場宎趙 startday = (Spinner) findViewById(R.id.startday); adapter3 = new ArrayAdapter<String>(this, android.R.layout.simple_spinner_item, dayoption31); adapter3.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item); startday.setAdapter(adapter3); startday.setVisibility(View.VISIBLE); startday.setOnItemSelectedListener(new SpinnerSelectedListener3());
// 賦旰 爛爺狟嶺蹈桶 endyear = (Spinner) findViewById(R.id.endyear); adapter4 = new ArrayAdapter<String>(this, android.R.layout.simple_spinner_item, yearoption); adapter4.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item); endyear.setAdapter(adapter4); endyear.setVisibility(View.VISIBLE); endyear.setOnItemSelectedListener(new SpinnerSelectedListener4()); // 賦旰堎爺狟懂蹈桶 endmonth = (Spinner) findViewById(R.id.endmonth); adapter5 = new ArrayAdapter<String>(this, android.R.layout.simple_spinner_item, monthoption); adapter5.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item); endmonth.setAdapter(adapter5); endmonth.setVisibility(View.VISIBLE); endmonth.setOnItemSelectedListener(new SpinnerSelectedListener5()); // 賦旰狟嶺蹈桶場宎趙 endday = (Spinner) findViewById(R.id.endday); adapter6 = new ArrayAdapter<String>(this, android.R.layout.simple_spinner_item, dayoption31); adapter6.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item); endday.setAdapter(adapter3); endday.setVisibility(View.VISIBLE); endday.setOnItemSelectedListener(new SpinnerSelectedListener6());
inquirydata = (Button) findViewById(R.id.inquirydata); inquirypicture = (Button) findViewById(R.id.inquirypicture); inquirydata.setOnClickListener(new Buttonlistener1()); inquirypicture.setOnClickListener(new Buttonlistener2());
}
class SpinnerSelectedListener1 implements OnItemSelectedListener {
public void onItemSelected(AdapterView<?> arg0, View arg1, int arg2, long arg3) { sy = adapter1.getItem(arg2); // if(adapter1.getItem(arg2).equals("2016")) // System.out.println(adapter1.getItem(arg2));
}
public void onNothingSelected(AdapterView<?> arg0) { } }
class SpinnerSelectedListener2 implements OnItemSelectedListener {
public void onItemSelected(AdapterView<?> arg0, View arg1, int arg2, long arg3) { sm = adapter2.getItem(arg2); if (adapter2.getItem(arg2).equals("01") || adapter2.getItem(arg2).equals("03") || adapter2.getItem(arg2).equals("05") || adapter2.getItem(arg2).equals("07") || adapter2.getItem(arg2).equals("08") || adapter2.getItem(arg2).equals("10") || adapter2.getItem(arg2).equals("12")) monthmark = 31; else if (adapter2.getItem(arg2).equals("04") || adapter2.getItem(arg2).equals("06") || adapter2.getItem(arg2).equals("09") || adapter2.getItem(arg2).equals("11")) monthmark = 30; else if (adapter1.getItem(arg2).equals("2016") || adapter2.getItem(arg2).equals("02")) monthmark = 29; else monthmark = 28; }
public void onNothingSelected(AdapterView<?> arg0) { } }
class SpinnerSelectedListener3 implements OnItemSelectedListener {
public void onItemSelected(AdapterView<?> arg0, View arg1, int arg2, long arg3) { sd = adapter3.getItem(arg2); }
public void onNothingSelected(AdapterView<?> arg0) { } }
class SpinnerSelectedListener4 implements OnItemSelectedListener {
public void onItemSelected(AdapterView<?> arg0, View arg1, int arg2, long arg3) { ey = adapter4.getItem(arg2); }
public void onNothingSelected(AdapterView<?> arg0) { } }
class SpinnerSelectedListener5 implements OnItemSelectedListener {
public void onItemSelected(AdapterView<?> arg0, View arg1, int arg2, long arg3) { em = adapter5.getItem(arg2);
}
public void onNothingSelected(AdapterView<?> arg0) { } }
class SpinnerSelectedListener6 implements OnItemSelectedListener {
public void onItemSelected(AdapterView<?> arg0, View arg1, int arg2, long arg3) {
ed = adapter6.getItem(arg2); }
public void onNothingSelected(AdapterView<?> arg0) { } }
class Buttonlistener1 implements View.OnClickListener { @Override public void onClick(View v) {
sh = String.valueOf(starttp.getCurrentHour()); smm = String.valueOf(starttp.getCurrentMinute()); eh = String.valueOf(endtp.getCurrentHour()); emm = String.valueOf(endtp.getCurrentMinute());
start = sy + "-" + sm + "-" + sd + " " + sh + ":" + smm; end = ey + "-" + em + "-" + ed + " " + eh + ":" + emm; interval = "0";
Intent intent = new Intent();
intent.putExtra("username", confirmedname); intent.putExtra("greenhouse", greenhouse); intent.putExtra("node", node); intent.putExtra("device", device); intent.putExtra("start", start); intent.putExtra("end", end); intent.putExtra("interval", interval); intent.setClass(Inquirybydate.this, Listresult.class); Inquirybydate.this.startActivity(intent);
}
}
class Buttonlistener2 implements View.OnClickListener { @Override public void onClick(View v) {
sh = String.valueOf(starttp.getCurrentHour()); smm = String.valueOf(starttp.getCurrentMinute()); eh = String.valueOf(endtp.getCurrentHour()); emm = String.valueOf(endtp.getCurrentMinute());
start = sy + "-" + sm + "-" + sd + " " + sh + ":" + smm; end = ey + "-" + em + "-" + ed + " " + eh + ":" + emm; interval = "0";
System.out.println(start); Intent intent = new Intent();
intent.putExtra("username", confirmedname); intent.putExtra("greenhouse", greenhouse); intent.putExtra("node", node); intent.putExtra("device", device); intent.putExtra("start", start); intent.putExtra("end", end); intent.putExtra("interval", interval); intent.setClass(Inquirybydate.this, Line.class); Inquirybydate.this.startActivity(intent);
}
}
}
*****
interface:
package com.example.iot;
import org.ksoap2.SoapEnvelope; import org.ksoap2.serialization.SoapObject; import org.ksoap2.serialization.SoapSerializationEnvelope; import org.ksoap2.transport.HttpTransportSE;
import android.widget.ListView;
public class Inquirybydateweb implements Runnable { public ListView list; public int length; public SoapObject result; public String[] listcontent; private static final String namespace = "http://category"; private static final String methodname = "category"; private static final String URL = "http://202.194.26.192:8080/category/services/category"; public boolean tag = true; private String username = null; private String greenhouse = null; private String node = null; private String device = null; private String starttime = null; private String endtime = null; private String interval = null;
Inquirybydateweb(String username1, String greenhouse1, String node1, String device1, String starttime1, String endtime1, String interval1) { this.username = username1; this.greenhouse = greenhouse1; this.node = node1; this.device = device1; this.starttime = starttime1; this.endtime = endtime1; this.interval = interval1; }
public void run() { SoapObject rpc = new SoapObject(namespace, methodname); rpc.addProperty("userName", username); rpc.addProperty("greenHouse", greenhouse); rpc.addProperty("node", node); rpc.addProperty("device", device); rpc.addProperty("starttime", starttime); rpc.addProperty("endtime", endtime); rpc.addProperty("interval", interval);
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope( SoapEnvelope.VER10); envelope.bodyOut = rpc; envelope.dotNet = true; envelope.setOutputSoapObject(rpc);
HttpTransportSE transport = new HttpTransportSE(URL);
try {
transport.call(null, envelope); if (envelope.getResponse() != null) { result = (SoapObject) envelope.getResponse(); length = result.getPropertyCount(); } else length=0; } catch (Exception e) { e.printStackTrace(); }
System.out.println(length); System.out.println(length); System.out.println(length); System.out.println(length); listcontent = new String[length]; if (length > 0) { for (int i = 0; i <= length - 1; i++) { listcontent[i] = result.getProperty(i).toString();
}
} tag = false; }
}
#6.spectrum searching/ display/ intercace
page:
package com.example.iot;
import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.AdapterView.OnItemSelectedListener; import android.widget.ArrayAdapter; import android.widget.Button; import android.widget.Spinner; import android.widget.TextView; import android.widget.TimePicker;
public class SpectrumInquiry extends Activity {
private String confirmedname; private String greenhouse; private String node;
private String[] yearoption = { "2015", "2016", "2017" }; private String[] monthoption = { "01", "02", "03", "04", "05", "06", "07", "08", "09", "10", "11", "12" }; private String[] dayoption31 = { "01", "02", "03", "04", "05", "06", "07", "08", "09", "10", "11", "12", "13", "14", "15", "16", "17", "18", "19", "20", "21", "22", "23", "24", "25", "26", "27", "28", "29", "30", "31" }; public static ArrayAdapter<String> adapter11; public static ArrayAdapter<String> adapter22; public static ArrayAdapter<String> adapter33;
private Spinner year; private Spinner month; private Spinner day; private TimePicker tp;
private String y; private String m; private String d; private String h; private String mm; private String time;
private TextView spectruminquirytitle; private Button inquiry;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.spectruminquiry); spectruminquirytitle = (TextView) findViewById(R.id.spectruminquirytitle); inquiry = (Button) findViewById(R.id.inquiry); tp = (TimePicker) findViewById(R.id.tp); tp.setIs24HourView(true);
Intent intent = getIntent(); this.confirmedname = intent.getStringExtra("username"); this.greenhouse = intent.getStringExtra("greenhouse"); this.node = intent.getStringExtra("node"); spectruminquirytitle.setText("覜眭誹萸"+node+"瘍腔" + "腔嫖杅擂"); inquiry.setOnClickListener(new Buttonlistener());
// 羲宎 爛爺狟嶺蹈桶 year = (Spinner) findViewById(R.id.year); adapter11 = new ArrayAdapter<String>(this, android.R.layout.simple_spinner_item, yearoption); adapter11 .setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item); year.setAdapter(adapter11); year.setVisibility(View.VISIBLE); year.setOnItemSelectedListener(new SpinnerSelectedListener11()); // 羲宎堎爺狟懂蹈桶 month = (Spinner) findViewById(R.id.month); adapter22 = new ArrayAdapter<String>(this, android.R.layout.simple_spinner_item, monthoption); adapter22 .setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item); month.setAdapter(adapter22); month.setVisibility(View.VISIBLE); month.setOnItemSelectedListener(new SpinnerSelectedListener22()); // 羲宎狟嶺蹈桶場宎趙 day = (Spinner) findViewById(R.id.day); adapter33 = new ArrayAdapter<String>(this, android.R.layout.simple_spinner_item, dayoption31); adapter33 .setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item); day.setAdapter(adapter33); day.setVisibility(View.VISIBLE); day.setOnItemSelectedListener(new SpinnerSelectedListener33());
}
class SpinnerSelectedListener11 implements OnItemSelectedListener {
public void onItemSelected(AdapterView<?> arg0, View arg1, int arg2, long arg3) { y = adapter11.getItem(arg2);
}
public void onNothingSelected(AdapterView<?> arg0) { } }
class SpinnerSelectedListener22 implements OnItemSelectedListener {
public void onItemSelected(AdapterView<?> arg0, View arg1, int arg2, long arg3) { m = adapter22.getItem(arg2);
}
public void onNothingSelected(AdapterView<?> arg0) { } }
class SpinnerSelectedListener33 implements OnItemSelectedListener {
public void onItemSelected(AdapterView<?> arg0, View arg1, int arg2, long arg3) { d = adapter33.getItem(arg2); }
public void onNothingSelected(AdapterView<?> arg0) { } }
class Buttonlistener implements View.OnClickListener { @Override public void onClick(View v) {
h = String.valueOf(tp.getCurrentHour()); mm = String.valueOf(tp.getCurrentMinute());
time = y + "-" + m + "-" + d + " " + h + ":" + mm;
Intent intent = new Intent();
intent.putExtra("username", confirmedname); intent.putExtra("greenhouse", greenhouse); intent.putExtra("node", node); intent.putExtra("time", time);
intent.setClass(SpectrumInquiry.this, Spectrumtimerangeshow.class); SpectrumInquiry.this.startActivity(intent);
}
}
}
*****
display is shown in #0
******
interface:
package com.example.iot;
import org.ksoap2.SoapEnvelope; import org.ksoap2.serialization.SoapObject; import org.ksoap2.serialization.SoapSerializationEnvelope; import org.ksoap2.transport.HttpTransportSE;
import android.widget.ListView;
public class Spectrumtimerangeshowweb implements Runnable { public ListView list; public int length; public SoapObject result; public String[] listcontent; private static final String namespace = "http://spectrumdatalist"; private static final String methodname = "spectrumdatalist"; private static final String URL = "http://202.194.26.192:8080/spectrumdatalist/services/spectrumdatalist"; public boolean tag = true; private String username = null; private String greenhouse = null; private String node = null; private String time = null;
Spectrumtimerangeshowweb(String username1, String greenhouse1, String node1, String time1) { this.username = username1; this.greenhouse = greenhouse1; this.node = node1; this.time = time1; }
public void run() { SoapObject rpc = new SoapObject(namespace, methodname); rpc.addProperty("userName", username); rpc.addProperty("greenHouse", greenhouse); rpc.addProperty("node", node); rpc.addProperty("time", time);
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope( SoapEnvelope.VER10); envelope.bodyOut = rpc; envelope.dotNet = true; envelope.setOutputSoapObject(rpc);
HttpTransportSE transport = new HttpTransportSE(URL);
try {
transport.call(null, envelope); if (envelope.getResponse() != null) { result = (SoapObject) envelope.getResponse(); length = result.getPropertyCount();
} else length=0; } catch (Exception e) { e.printStackTrace(); }
listcontent = new String[length]; if (length > 0) { for (int i = 0; i <= length - 1; i++) { listcontent[i] = result.getProperty(i).toString();
}
} tag = false; }
}